ConsoleTextFormat: Formatting Console App Text - Part 2
The previous post covered ConsoleTextFormat. a simple static class library facilitating .NET Console app text formatting in Console.Write()
and Console.WriteLine()
statements without explicitly using escape codes. This post adds some UI methods for displaying headings, prompts and menus (just added) using this formatting.
This is the second post with respect to the ConsoleTextFormat library. The first post covered the implicit use of escape strings for formatting Console app text. This adds some capabilities for headings, prompts and menus. Note that these have been moved into a separate class Layout
from the Fmt
class with the latest release of the package.
Links
- GitHub Repository: djaus2/ConsoleTextFormat
- Nuget Package: ConsoleTextFormat
About
Headings
Fmt also has 3 other options:
Heading()
- Clear the screen
- Writes the supplied string on the top line:
- Double spaced
- Font Bold and Colored (White default)
- Background (Black default)
- Format cleared at end
RainbowHeading()
- Clear the screen
- Write the supplied string on the top line:
- Double spaced
- Background (Black default)
- Font Bold and alternating colors (not = to background)
- Format cleared at end.
Info()
- Write on one line
- Two strings supplied.
- First written in bold and first color (default blue) supplied.
- Second written following in second color default yellow) not bold.
- Format cleared at end
Prompt()
= As per Info but no newline at end.- Basis for getting user response.
Example Headings Console Code
using B = ConsoleTextFormat.Fmt.Bold;
using F = ConsoleTextFormat.Fmt;
namespace ConsoleApp9
{
using System;
using ConsoleTextFormat;
internal class Program
{
static void Main(string[] args)
{
F.Heading("Hello, World!",Fmt.Col.yellow, Fmt.Col.blue);
F.RainbowHeading("Hello, World!", Fmt.Col.yellow);
F.Info("This is a topic:", "Information"); ;
}
}
}
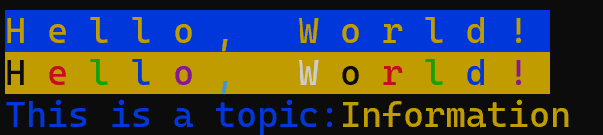
Console app output
“Press to Continue” Prompt
Press2Continue()
- Displays “Press any key to continue”
- Optional Pre and Post test to add on same line.
- Waits for any key press.
Example Press Any Key Console Code
Layout.Press2Continue();
Layout.Press2Continue("Wait for completion");
Layout.Press2Continue("","when ready.");
Layout.Press2Continue("Wait for completion", "when ready.");

Console app output
Menus
SelectEnum<T>()
- Displays a list of all enums from an enum type
- Prompts for selection using a single key _(No return required).
- Has optional [Q] for quit.
- Is constructed as layers of methods.
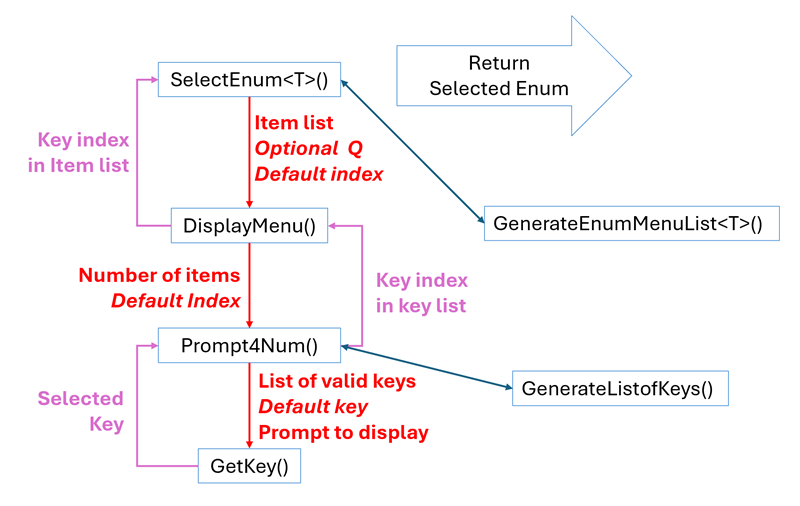
Blockly Start options
Notes
SelectEnums<T>()
gets the specified enum type literals names as a list of string and passes it toDisplayMenu()
.- With enums that have underscore in name, that is replaced by a space
- For example, ```First_Name`` is listed as “First Name”
- If the optional quit (lowercase) parameter (default is false) is true then ‘Q’ is added to the list.
- Note that it is passed back in the Quit (uppercase) parameter.
- With enums that have underscore in name, that is replaced by a space
DisplayMenu()
is the method that actually displays the list of items, each with an index from 1 to select.Prompt4Num()
generates the appropriate prompt to display and passes it toGetKey()
- Converts the returned char to an index and returns that.
- Nb: ‘A’ is returned as 10 etc.
- Converts the returned char to an index and returns that.
GetKey()
handlesConsole.ReadKey()
and returns the selected key character- Where more than 9 items in list, case insensitive keys ‘A’,’B’,’C’ … are displayed for 10,11,12 … for selection. The corresponding 10,11,12 … is returned if a ‘A’,’B,’C’ … is chosen.
DisplayMenu()
can be used directly with any List of string to generate a menu and return the selected item index as an int.
Example Menu Console Code
var lst = Layout.GenerateEnumMenuList<Animals>();
foreach (var item in lst)
{
Console.WriteLine(item);
}
char ch = Layout.Prompt4Ch("Select an number", '2', new List<char> { '1', '2', '3' });
Console.WriteLine($"{ch} selected");
int menuResp = Layout.Prompt4Num(2, 4, true);
if (menuResp < 0) return; //Quit
menuResp = Layout.Prompt4Num(2, 12, true);
if (menuResp < 0) return; //Quit
bool quit = false;
Animals animal = Layout.SelectEnum<Animals>(2, ref quit, true);
if (quit) return; //Quit
Console.WriteLine($"Selected: {animal}");
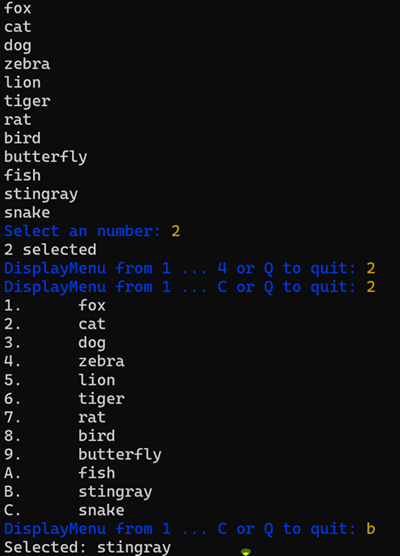
Console app output
There are also some background and foreground color options with the menus.
Updates
- 2021-09-16: Moved Headings etc to Layout class.
- 2021-09-16: Added
Layout.Press2Continue()
- 2021-09-16: Added
Layout.SelewctEnum<T>())
plus others as above.
Topic | Subtopic | |
This Category Links | ||
Category: | Coding Index: | Coding |
Next: > | ConsoleTextFormat | Menus |
< Prev: | ConsoleTextFormat | Formatting Console App Text - Part 1 |

