ConsoleTextFormat: Formatting Console App Text
A simple static class library facilitating
.NET Console app text formatting in Console.Write()
and Console.WriteLine()
statements without explicitly using escape codes.
Links
- GitHub Repository: djaus2/ConsoleTextFormat
- Nuget Package: ConsoleTextFormat
About
Whilst there are some Console class methods for setting text and background colors, there are no methods for setting text attributes such as bold, underline, etc. This library provides a simple way to do this.
This library enables inline code (that is, with in a string) to change text format when
writing to the console using Console.Write() and Console.WriteLine()
methods.
The class entities have been made a cryptic as possible whilst maintaining interpretation upon inspection. The strings so used are not escaped, but are interpreted by the library.
String Interpolation is used to embed the format strings in the text to be written to the console.
The format strings are at 2 levels.
- Quite explicit e.g.
{Fmt.fg(Fmt.Col.yellow)}
- Cryptic e.g.
{F.fgYel}
Both of the code segments above will produce the same result. The cryptic version requires the using statements as in the following code to alias the class names.
Begin and End
HTML code has begin and end tags. ConsoleTextFormat is similarly construed. If, for example a bold font is used, then that will continue in all Console.Writes until terminated.
Console.WriteLine($"{Fmt.b}Hello{Fmt._b}");
Console.WriteLine($"{ul}World!{_ul}");
This is similar to the following HTML code:
<b>Hello!</b>
<u>World!</u>
There is though a reset to default string, Fmt.clear
:
Console.WriteLine($"{Fmt.b}Hello{Fmt.clear}");
Consider the following HTML code:
<b><u><font color="blue">Hello</font></u></b>
The equivalent in ConsoleTextFormat can be terminated more simply:
Console.WriteLine($"{Fmt.b}{ul}{Fmt.fg(Fmt.Col.blue)}Hello{Fmt.clear}");
Under the hood
Link: Background on MS Learn: Console Virtual Terminal Sequences See Text Formatting
The text formatting in console text is specified by the following table:
Sequence | Code | Description | Behavior |
---|---|---|---|
ESC [ |
SGR | Set Graphics Rendition | Set the format of the screen and text as specified by <n> |
This manifests in C# code as \u001b[<n>m
. For example:
/// <summary>
/// Bold
/// </summary>
public const string b = "\u001b[1m";
/// <summary>
/// Not Bold
/// </summary>
public const string _b = "\u001b[22m";
And so in an interpolated string (starts with a dollar sign) you insert {Fmt.b} … {Fmt._b} to make part of the string bold.
Note the coded escaped strings. The values 30+ for foreground colors 30,31 … (black, red …) and 40,41 … for background colors. These values are listed in the MS Learn link above. Note there are also other formats,all starting at black
- 30 black foreground
- 40 black background
- 90 black bold-foreground
- 100 black bold-background
Colors
The package has an enum list of colors that are used for setting background and text foreground colors:
/// <summary>
/// Foreground/Background Format colors
/// </summary>
public enum Col { black = 0, red, green, yellow, blue, magenta, cyan, white };
These are used in foreground and background color specifications:
public static string fg(Col clr) { return $"\u001b[{30 + (int)clr}m"; }
public static string bg(Col clr) { return $"\u001b[{40 + (int)clr}m"; }
… where clr is one of the Col enums. For example:
{fg(yellow)}
{bg(white)}
The colors have been separately enumerated with color names cut to 2 letters to make the color insertions shorter but a bit cryptic. For example the above can also be inserted as:
{fgYel}
{bgWhi}
For readability the colors start with a capital letter.
Headings
Fmt also has 3 other options:
- Heading
- Clear the screen
- Write the supplied string on the top line:
- Double spaced
- Font Bold and Colored (White default)
- Background (Black default)
- Format cleared at end
- RainbowHeading
- Clear the screen
- Write the supplied string on the top line:
- Double spaced
- Background (Black default)
- Font Bold and alternating colors (not = to background)
- Format cleared at end.
- Info
- Write on one line
- Two strings supplied.
- First written in bold and first color (default blue) supplied.
- Second written following in second color default yellow) not bold.
- Format cleared at end
Alias names
T o simplify use you can add some using statements such as
using B = ConsoleTextFormat.Fmt.Bold;
using F = ConsoleTextFormat.Fmt;
You then can use B.
and F.
as below:
Example Console Code
using B = ConsoleTextFormat.Fmt.Bold;
using F = ConsoleTextFormat.Fmt;
namespace ConsoleApp9
{
using System;
using ConsoleTextFormat;
internal class Program
{
static void Main(string[] args)
{
Fmt.Heading("Hello, World!",Fmt.Col.yellow, Fmt.Col.blue);
Fmt.RainbowHeading("Hello, World!", Fmt.Col.yellow);
Fmt.Info("This is a topic:", "Information");
Console.WriteLine($"{Fmt.bg(Fmt.Col.white)}Hello, {Fmt.b}World!{Fmt._b}{Fmt.clear}");
Console.WriteLine($"{Fmt.fg(Fmt.Col.yellow)}Hello, {Fmt.b}World!{Fmt._b}{Fmt.clear}");
Console.WriteLine($"{Fmt.fg(Fmt.Col.blue)}Hello, {Fmt.clear}World!");
Console.WriteLine($"{B.fgRed}{B.bgCya}Hello, {Fmt.clear}World!");
Console.WriteLine($"{F.fgRed}{F.bgCya}Hello, {Fmt.clear}World!");
}
}
}
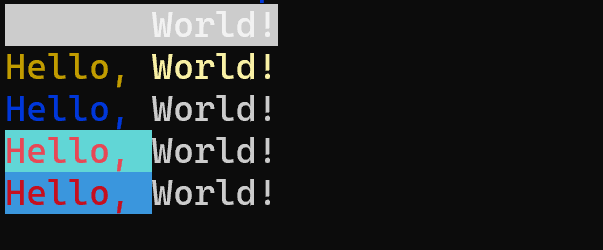
Blockly Start options
Console app output
Updates
- 2021-09-07: Added
Fmt.Heading(heading)
andFmt.RainbowHeading(heading)
methods to format text as headings. - 2021-09-07: Added
Fmt.Info(topic,info)
added.
Topic | Subtopic | |
< Prev: | Softata | Blockly Session |
This Category Links | ||
Category: | Coding Index: | Coding |
< Prev: | C++ Coding | const char * data type |

